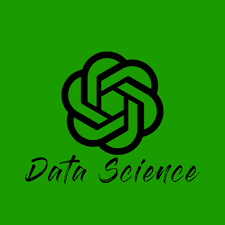
Advanced prompts for data science projects
Here are some advanced prompts for data science projects:
Prompt: "I have a large dataset, and I'm interested in implementing feature selection techniques to improve my model performance. Can you suggest some methods and explain how to use them?"
Example completion: "Feature selection is a crucial step to improve model performance and reduce overfitting. There are various techniques you can consider. One popular method is Recursive Feature Elimination (RFE),which recursively eliminates features by fitting the model multiple times. You can use scikit-learn's RFE implementation, as shown in the example below:"
from sklearn.feature_selection import RFE
from sklearn.linear_model import LogisticRegression
# Assuming 'X' is your feature matrix and 'y' is the target variable
estimator = LogisticRegression()
rfe = RFE(estimator, n_features_to_select=5) # Select the desired number of features
X_selected = rfe.fit_transform(X, y)
"Another technique is SelectKBest, which selects the top K features based on statistical tests. Here's an example using SelectKBest with chi-square test for feature selection:"
· from sklearn.feature_selection import SelectKBest, chi2
# Assuming 'X' is your feature matrix and 'y' is the target variable
selector = SelectKBest(score_func=chi2, k=10) # Select the desired number of features
X_selected = selector.fit_transform(X, y)
Prompt: "I want to optimize the hyperparameters of my machine learning model. What are some techniques I can use for hyperparameter tuning?"
Example completion: "Hyperparameter tuning is essential to optimize model performance. One popular technique is Grid Search, where you specify a grid of hyperparameters and evaluate the model's performance on each combination. Scikit-learn provides a convenient implementation of Grid Search, as shown below:"
from sklearn.model_selection import GridSearchCV
from sklearn.ensemble import RandomForestClassifier
# Assuming 'X' is your feature matrix and 'y' is the target variable
param_grid = {'n_estimators': [100, 200, 300], 'max_depth': [None, 5, 10]}
model = RandomForestClassifier()
grid_search = GridSearchCV(estimator=model, param_grid=param_grid, cv=5)
grid_search.fit(X, y)
best_params = grid_search.best_params_
best_model = grid_search.best_estimator_
Another technique is Randomized Search, which randomly samples from a distribution of hyperparameters. This can be beneficial when the search space is large.
Feel free to modify these prompts based on the specific algorithms, techniques, or libraries you're using in your project.
Article By:-
Er. Sumit Malhotra
Assistant Professor
Chandigarh University
Gharuan (Mohali),Punjab
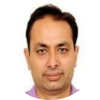
Article by Sumit Malhotra
Published 01 Apr 2024