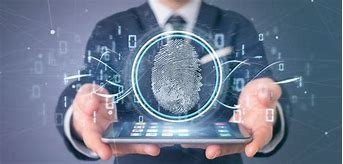
JavaAuthenticationPart1
Web Applications
In a web application using Java Servlets, the LoginServlet
and LogoutServlet
handle the login and logout functionality respectively. When a user submits their login credentials via a POST request to the /login
endpoint, the LoginServlet
extracts the username
and password
parameters from the request. It then checks if these credentials match hardcoded values ("admin" and "password"). If they do, it creates a new session or retrieves the existing session using request.getSession()
, stores the username in the session using session.setAttribute("user", username)
, and redirects the user to a welcome page (welcome.jsp
). If the credentials are invalid, the user is redirected back to the login page (login.jsp
). The LogoutServlet
, mapped to the /logout
endpoint, retrieves the current session using request.getSession(false)
, invalidates it to log the user out, and then redirects to the login page (login.jsp
).
Code
Using Servlets and Sessions
LoginServlet.java
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
@WebServlet("/login")
public class LoginServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String username = request.getParameter("username");
String password = request.getParameter("password");
if ("admin".equals(username) && "password".equals(password)) {
HttpSession session = request.getSession();
session.setAttribute("user", username);
response.sendRedirect("welcome.jsp");
}else {
response.sendRedirect("login.jsp");
}
}
}
LogoutServlet.java
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
@WebServlet("/logout")
public class LogoutServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
HttpSession session = request.getSession(false);
if (session != null) {
session.invalidate();
}
response.sendRedirect("login.jsp");
}
}
For more details find part 2 in the blog,next week.
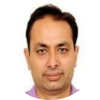
Article by Sumit Malhotra
Published 03 Dec 2023