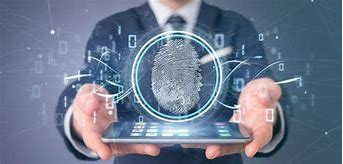
JavaAuthenticationPart2
Desktop Applications
Using JavaFX
In a JavaFX desktop application, the LoginController
class manages the login and logout functionality. The FXML annotations (@FXML
) are used to bind UI components, such as usernameField
and passwordField
, to their respective fields in the controller. When the login
method is called, it retrieves the text entered in the username and password fields. It then checks these values against hardcoded credentials ("admin" and "password"). If the credentials are valid, the application would navigate to the main application window (this part is typically implemented by loading a new scene or stage). If the credentials are invalid, an error alert is displayed to the user using the Alert
class. The logout
method would handle closing the main application window and showing the login window, though its implementation is not detailed here.
LoginController.java
import javafx.fxml.FXML;
import javafx.scene.control.TextField;
import javafx.scene.control.PasswordField;
import javafx.scene.control.Alert;
import javafx.scene.control.Alert.AlertType;
public class LoginController {
@FXML
private TextField usernameField;
@FXML
private PasswordField passwordField;
public void login() {
String username = usernameField.getText();
String password = passwordField.getText();
if ("admin".equals(username) && "password".equals(password)) {
// Navigate to the main application window
}else {
Alert alert = new Alert(AlertType.ERROR);
alert.setContentText("Invalid credentials");
alert.show();
}
}
public void logout() {
// Handle logout (e.g., close the main window and show the login window)
}
}
For more details find part 3 in the blog,next week.
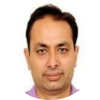
Article by Sumit Malhotra
Published 10 Dec 2023