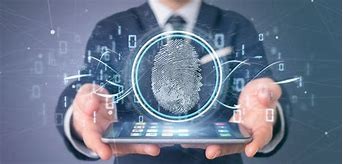
JavaAuthenticationPart5
Using JWT (JSON Web Tokens) for REST APIs
JwtAuthenticationFilter.java
import import import import import import import import import import import import public
|
This class extends UsernamePasswordAuthenticationFilter
and customizes it to use JWT tokens for authentication. When a login request is made, the attemptAuthentication
method is triggered. It extracts the username and password from the HTTP request and creates an UsernamePasswordAuthenticationToken
which is then authenticated by the AuthenticationManager
. If the authentication is successful, the successfulAuthentication
method is called. This method generates a JWT token using Jwts.builder()
. The token contains the username as the subject and an expiration date (10 days in this case). The token is signed using the HS512 algorithm and a secret key. Finally, the token is added to the HTTP response header. This setup allows stateless authentication, where the token is sent with each request to verify the user’s identity, eliminating the need for server-side session management.
The next Part will be released in the new year on 7 January 2024.
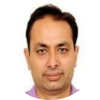
Article by Sumit Malhotra
Published 31 Dec 2023