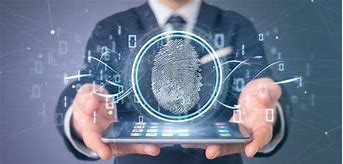
JavaAuthenticationPart8
Using JAAS (Java Authentication and Authorization Service)
JAASLogin.java
java
Copy code
import javax.security.auth.login.LoginContext;
import javax.security.auth.login.LoginException;
public class JAASLogin {
public static void main(String[] args) {
System.setProperty("java.security.auth.login.config", "path/to/jaas.config");
try {
LoginContext lc = new LoginContext("example", new MyCallbackHandler());
lc.login();
System.out.println("Authentication successful");
}catch (LoginException e) {
e.printStackTrace();
}
}
}
jaas.config
properties
Copy code
example {
com.sun.security.auth.module.LdapLoginModule REQUIRED
userProvider="ldap://localhost:389/o=example"
authIdentity="uid={USERNAME},ou=People,o=example"
userPassword="secret";
};
Explanation:
JAAS provides a standard way to handle authentication and authorization in Java applications. The JAASLogin class sets the system property java.security.auth.login.config to point to the JAAS configuration file. This file (jaas.config) specifies the login module to be used (in this case, LdapLoginModule for LDAP authentication) and its configuration parameters, including the LDAP server URL, authentication identity pattern, and user password. The LoginContext class is instantiated with the name of the login configuration (example) and a custom callback handler (MyCallbackHandler) to handle user input for credentials. The login() method is called to authenticate the user. If authentication is successful, a success message is printed; otherwise, an exception is caught and its stack trace is printed. This setup provides a flexible and extensible framework for integrating various authentication mechanisms into a Java application.
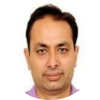
Article by Sumit Malhotra
Published 21 Jan 2024